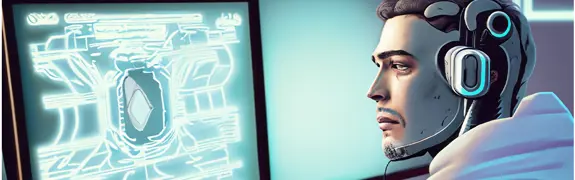
using AI to build software and how to avoid the race to the average
Using AI to build software can result in a slippery slope towards developing average code.
PLEASE ACCEPT OUR. COOKIE POLICY
In my experience, getting content indexed by the Bing Search Engine can be a frustrating and difficult experience, especially when compared to Google. In addition, even when your content passes all the Bing tests, getting new content into the Bing Index can take a while.
Microsoft doesn't make it crystal clear what the best way of getting content into the Bing Index is, but it does currently seem to recommend using the WebMaster API. And, from what I can tell, using the API seems to speed up the process of getting your content listed dramatically. Best of all you can easily automate it.
This article describes how to use .Net 5 and C# to connect to the Bing Webmaster Tools API and post Urls to it.
To get your website content indexed you can add a sitemap file (usually "sitemap.xml" at the root of your site) which Bing can then use to get a list of your urls to crawl. In the worst case scenario you may have to update your sitemap manually, which is obviously a bit tedious and error prone. In the best case you can generate your sitemap dynamically (whenever the url is requested).
However, even if you generate your sitemap dynamically you still need to wait for Bing to look at your sitemap. If you are just getting your site started it is likely your site will not be a top priority for Bing. Therefore, after you have created some new content you might have to wait a while before Bing crawls your site again.
If you don't want to wait for Bing to look at your sitemap.xml you can submit Urls manually by logging into Webmaster Tools and going to "Url Submission".
This is still a manual and error prone process though as you have to remember to go into Webmaster Tools every time you add a page or make a change to one.
The method recommended by Microsoft to get web content indexed quickly is to submit Urls directly to the WebMaster Tools API. So the most efficient way of incorporating this into your content publishing process is to automatically call the Webmaster Tools API each time you add or update a Url in your site using your CMS.
The rest of this article describes how to submit a Url to the Webmaster Tools API using C#. I am using .Net 5 and therefore C# 9 but this code will work on much older versions of .Net and C# as well.
Once you have access to Webmaster Tools you can get an API Key by clicking on the Settings cog and requesting one.
Since I publish content from a web application I will add the key into my config by adding the following line to my "appsettings.json" file:
"BingAPIKey": "your-api-key-from-webmaster-tools",
We need to create a class to hold the url of your site and the url of the content that is being added or updated. Add a new class to your application that looks like the following (obviously you will need to make changes to the constructor to match your own requirements):
public class WebMasterAPIUrlModel
{
public string siteUrl { get; set; }
public string url { get; set; }
public WebMasterAPIUrlModel(string newurl)
{
siteUrl = "https://yoursite.com";
url = "https://yoursite.com/blog/" + newurl;
}
}
Now we need to create a method to connect to the Bing Webmaster Tools API using HttpClient, provide it with your BingAPIKey and pass an object containing your site url and the new or updated url to add to the Bing Index. To do this add the following method which will get called when you add a new url or update an existing url:
private async Task PostUrlToBing(string newUrl)
{
string bingNewUrlUri = "https://ssl.bing.com/webmaster/api.svc/json/SubmitUrl?apiKey=" + _configuration["BingAPIKey"];
HttpClient httpClient = new HttpClient();
var webMasterApiUrlObj = new WebMasterAPIUrlModel(newUrl);
var postRequest = new HttpRequestMessage(HttpMethod.Post, bingNewUrlUri)
{
Content = JsonContent.Create(webMasterApiUrlObj)
};
var postResponse = await httpClient.SendAsync(postRequest);
postResponse.EnsureSuccessStatusCode();
}
Finally your can hook the new code into your application. After your database, ORM or CMS system has sent a message back saying that the content has successfully been added or updated in your CMS database, we need to call the PostUrlToBing method we just created with the url that was added or updated. To do that add the following line into the relevent (add and update) methods of your application:
await PostUrlToBing(articleUrl);
That's it. Your application will now automatically submit Urls to Bing when a Url has been added in your CMS or notify Bing when the content in a Url has changed. The API sends back a message indicating success or otherwise but if you want to double check you can look in Webmaster Tools - Url Submission and the new url you have sent will be in the list :
After I added this particular Url to my website it took around 50 minutes before I could see that the Url had been added to the Bing Index. To do this I looked in Webmaster Tools - Url Inspection :
You can do a lot more with the API as well including getting traffic information for urls.
All the best.
Using AI to build software can result in a slippery slope towards developing average code.
Using DotNet 8 and Aspire With Azure Container Apps Makes Kubernetes Enabled Apps Incredibly Easy
Moving to a micro-services architecture can sometimes seem daunting due to the amount of technical choices to be made and the amount of planning involved. Often however, the migration can be made in manage-able steps. Here is one classic example.
Fast Endpoints have become very popular with .Net developers recently, but how much faster are they than regular Controller endpoints using .Net 8
GPT4 and other tools have good text summarisation capabilities but only Deep Mind is providing the real breakthroughs.
Managing Infrastructure State can be tricky in the cloud. AZTFY and Terraform can help with management