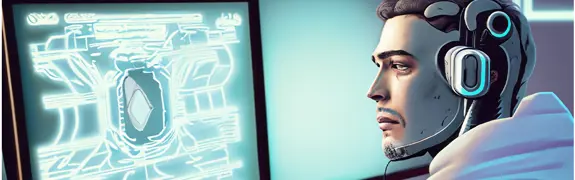
using AI to build software and how to avoid the race to the average
Using AI to build software can result in a slippery slope towards developing average code.
PLEASE ACCEPT OUR. COOKIE POLICY
In a python project recently we added linting tools to the pipeline to try to catch poor coding practice. Most of the "errors" that the linting tools highlighted were things like "does not conform to snake case" or "too many characters (more than 100) in line" .
In addition however, some real problems were highlighted. One was "too many arguments". After Googling this I took the suggestion to group any related arguments together in a class. Since the parameters were all related I created a new class with just an init method and the parameters in the constructor which were passed to properties on the class.
This refactoring however, generated a new error from pylint which was class uses . The new recommendation was that since the new class is just used to store data then I should use the @dataclass decorator.
The docs for this are here and implementing it is simple as in the following example:
@dataclass
class MyDataClass:
"""Class for keeping track of details."""name: str
age: int = 0
salary: float
Using the dataclass decorator means the fields in the class get data types matching those passed in from the constructor. Under the hood some dunder methods are created to pass the data from the constructor to the fields of the class.
Using the @dataclass decorator reminds me of the record type in C#. In C# records are actually not a class but not a struct either but they are very similar to creating a class with the @dataclass decorator in Python.
Using AI to build software can result in a slippery slope towards developing average code.
Using DotNet 8 and Aspire With Azure Container Apps Makes Kubernetes Enabled Apps Incredibly Easy
Moving to a micro-services architecture can sometimes seem daunting due to the amount of technical choices to be made and the amount of planning involved. Often however, the migration can be made in manage-able steps. Here is one classic example.
Fast Endpoints have become very popular with .Net developers recently, but how much faster are they than regular Controller endpoints using .Net 8
GPT4 and other tools have good text summarisation capabilities but only Deep Mind is providing the real breakthroughs.
Managing Infrastructure State can be tricky in the cloud. AZTFY and Terraform can help with management