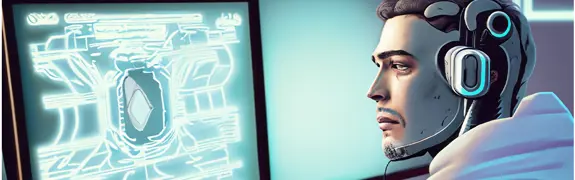
using AI to build software and how to avoid the race to the average
Using AI to build software can result in a slippery slope towards developing average code.
PLEASE ACCEPT OUR. COOKIE POLICY
In previous versions of Azure Functions, writing to Azure Blob Storage from an Azure Function was complicated. However with Version 3 of Azure Functions it couldn't be more simple. This article shows exactly how it is done using C#, DotNet Core 3 and Visual Studio 2019.
Even though it's simple it took me a bit of time to work out how to do it as the Microsoft explanation of this subject is a bit confusing. In addition, there are a lot of articles that explain how it is done in previous versions of Azure Functions, but I couldn't find any for how to do it in Version 3.
You should see the following:
Amend the signature of the function so that it includes an output binding to Storage, by replacing the existing code with the following:
[FunctionName("Function1")]
public static async TaskRun( [HttpTrigger(AuthorizationLevel.Function, "get", "post", Route = null)] HttpRequest req,
[Blob("outputdemo/{sys.utcnow}.txt", FileAccess.Write, Connection = "AzureWebJobsStorage")] Stream outputFile,
ILogger log)
Add the following code block just before the final return statement:
UnicodeEncoding uniencoding = new UnicodeEncoding();
string messageToWriteToFile = "Message from " + name;
byte[] output = uniencoding.GetBytes(messageToWriteToFile);
await outputFile.WriteAsync(output, 0, output.Length);
Test the function in the following way:
You should see the following returned from the browser
You should also be able to see the following in Azure Storage Explorer:
Now double click on the file and it should download. You should then be able to see the following if you view it in Notepad:
That's it. We wrote a file to Azure Storage using an Azure Function. You can download the finished project on github if you need to.
Using AI to build software can result in a slippery slope towards developing average code.
Using DotNet 8 and Aspire With Azure Container Apps Makes Kubernetes Enabled Apps Incredibly Easy
Moving to a micro-services architecture can sometimes seem daunting due to the amount of technical choices to be made and the amount of planning involved. Often however, the migration can be made in manage-able steps. Here is one classic example.
Fast Endpoints have become very popular with .Net developers recently, but how much faster are they than regular Controller endpoints using .Net 8
GPT4 and other tools have good text summarisation capabilities but only Deep Mind is providing the real breakthroughs.
Managing Infrastructure State can be tricky in the cloud. AZTFY and Terraform can help with management