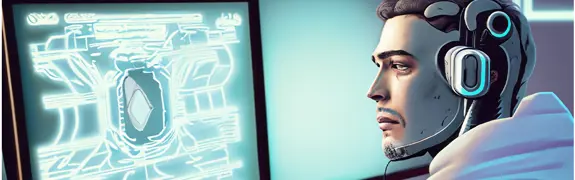
using AI to build software and how to avoid the race to the average
Using AI to build software can result in a slippery slope towards developing average code.
PLEASE ACCEPT OUR. COOKIE POLICY
This article is part of a series. We are on the way to connecting an Angular 9 App with a DotNetCore Web API. However, if we don't have CORS in our API we will see something like the following:
If you have Googled your way to this article you will be seeing something similar.
This article describes how to solve the problem.
Right mouse click on the project root and select "Manage Nuget Packages"
. In the "Browse" Tab type in "aspnetcore.cors".
Select "Microsoft.AspNetCore.Cors"
and then click "Install"
.
We will create a CORS policy called "OpenCORSPolicy". Like it's name suggests it's going to open up our API completely.
In "Startup.cs" add this line underneath the class statement:
readonly string CORSOpenPolicy = "OpenCORSPolicy";
Add this statement so that it is the first line of the "ConfigureServices" method:
services.AddCors(options =>
{
options.AddPolicy(name: CORSOpenPolicy,
builder =>
{
builder.WithOrigins("*").AllowAnyHeader()
.AllowAnyMethod();
});
});
Add the following line into the "Configure" method between the "UseRouting" and "UseAuthorization" statements:
app.UseRouting();
app.UseCors(CORSOpenPolicy);
app.UseAuthorization();
In each of the three controllers add the following line below the [ApiController] line:
[EnableCors("OpenCORSPolicy")]
We have added a very basic CORS policy to our Asp.Net Core Web API. If you got lost along the way, you can access the finished Git Repo here.
At this point it's important to note that CORS is not security. In fact a CORS policy relaxes security, and as the name of the policy suggests we have created a very relaxed policy. We now have an API that can be called by any client and this is a security hole. You will need to tighten this when your project gets out into the wild, or anywhere near it, so if you need more information here it is.
Using AI to build software can result in a slippery slope towards developing average code.
Using DotNet 8 and Aspire With Azure Container Apps Makes Kubernetes Enabled Apps Incredibly Easy
Moving to a micro-services architecture can sometimes seem daunting due to the amount of technical choices to be made and the amount of planning involved. Often however, the migration can be made in manage-able steps. Here is one classic example.
Fast Endpoints have become very popular with .Net developers recently, but how much faster are they than regular Controller endpoints using .Net 8
GPT4 and other tools have good text summarisation capabilities but only Deep Mind is providing the real breakthroughs.
Managing Infrastructure State can be tricky in the cloud. AZTFY and Terraform can help with management