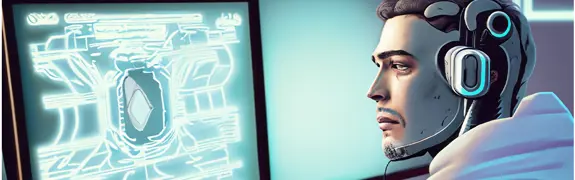
using AI to build software and how to avoid the race to the average
Using AI to build software can result in a slippery slope towards developing average code.
PLEASE ACCEPT OUR. COOKIE POLICY
This article is part of a series. If you want to start at exactly the same point you can quickly catch up by following this article. It won't take long.
As you probably know, Angular is component based. What you see on the screen at any point is the browser rendering the component that belongs to the view or the route that was chosen. A component is the combined result of the HTML (*.html), Typescript (*.ts) and CSS (*.ts) files that make it up. Finally, each top level component may display one or more child components as part of it's view.
In our App so far there is only one component which is called "app". It's files are created under the "src\app" folder. They are "app.component.ts", "app.component.html", "app.component.scss" and there is a testing file called "app.component.spec.ts".
We can now create some more components using the Angular CLI and see how we use Angular Routing to slot them into an App.
Incidentally, I use Visual Studio Code to edit Angular projects but there are lots of other good alternatives. In Visual Studio Code or your favorite editor open a folder or project at the root of the directory where you created your Angular App.
In the Angular CLI the full command to create a new component is:
"ng generate component \.
However, we can use a shorthand syntax to create what we need more speedily. The shorthand syntax to create a new component is:
"ng g c \"
I like to keep all components other than the app component in a directory called "components". Therefore, to create your components open a new terminal in Visual Studio Code and type or copy and paste in the following:
ng g c components\navmenu
ng g c components\funds-home
ng g c components\stocks-home
Angular CLI created the "components" directory under the app directory as it did not exist already, and the components were all created in that directory.
You have an Angular app with a few more components, however when you run your app using "ng serve" it will look exactly the same as it did. That's because your new components aren't included in any view at the moment. We can now start putting them in.
If you haven't already, open a browser at "http://localhost:4200" and watch the changes as you put them in. It's also a good way of trapping errors when they first occur. You will need to type "ng serve" again if you have closed your command prompt. You can do that from your terminal in Visual Studio Code.
We start editing by removing everything in the app.component.html file and replacing it with just the following:
Now you should only see the following on the screen in your browser:
In the browser our app component is now showing the navmenu at the top, which currently just says "navmenu works!" and then whatever has been defined using routing in the
We are going to have two routes - "stocks" and "funds". To define the routes we edit the "app.routing.module.ts" file which was created along with the rest of the App when we first ran the "ng new..." command.
In "app.routing.module.ts" you can see that the "routes" array is empty at the moment. To create the routes we need to add a line for each route to the "routes" array. Each line will define the name of the route and the component it should show when that route is called. It should now become:
const routes: Routes = [
{ path: 'stocks', component: StocksHomeComponent },
{ path: 'funds', component: FundsHomeComponent },
];
Now your editor will say you have an error. This is because we are referring to "StocksHomeComponent" and "FundsHomeComponent" which this file knows nothing about. Add the missing lines using ctl-. if you are in Visual Studio Code, or copy and paste the following into the top of the file:
import { StocksHomeComponent } from './components/stocks-home/stocks-home.component';
import { FundsHomeComponent } from './components/funds-home/funds-home.component';
We have created our routes but nothing will have changed in how our App looks. However, now that we have defined the routes we can refer to them from a menu, and when each item in the menu is clicked Angular will show the component that the route refers to. Let's copy and paste some code to create a very basic menu. Edit the "navmenu.component.html" file to show the following:
In the HTML code above you can see within each "a" tag we are providing the means of calling our routes. So this line:
will show "Funds" on the screen and when clicked will take the user to the "funds" route. Angular will then look at what we defined in our "app.routing.module.ts" file to get the component to show for the "funds" route, and therefore will show the "FundsHome" component.
You should now see the following on the screen when you click on the "Funds" menu option:
You can go back forth between the "funds" route and the "stocks" route. Note that the url in the browser changes and of course the display changes between "fund-home works!" and "stocks-home works!".
In case I have lost you, you can download the git repo from here
Using AI to build software can result in a slippery slope towards developing average code.
Using DotNet 8 and Aspire With Azure Container Apps Makes Kubernetes Enabled Apps Incredibly Easy
Moving to a micro-services architecture can sometimes seem daunting due to the amount of technical choices to be made and the amount of planning involved. Often however, the migration can be made in manage-able steps. Here is one classic example.
Fast Endpoints have become very popular with .Net developers recently, but how much faster are they than regular Controller endpoints using .Net 8
GPT4 and other tools have good text summarisation capabilities but only Deep Mind is providing the real breakthroughs.
Managing Infrastructure State can be tricky in the cloud. AZTFY and Terraform can help with management