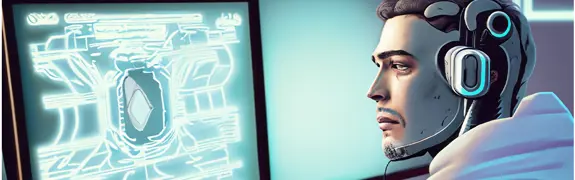
using AI to build software and how to avoid the race to the average
Using AI to build software can result in a slippery slope towards developing average code.
PLEASE ACCEPT OUR. COOKIE POLICY
This tutorial shows how to connect an AngularJS (client side JavaScript) application with an Entity Frameworks data source, within an Asp.Net MVC website. We use a "code first" approach to develop the Entity Frameworks data source.
This tutorial builds on a previous tutorial where we created a basic “Hello World” application in AngularJS within an MVC site. The development environment is Visual Studio 2013.
Firstly we need to build our Entity Framework data model. We will use a "code first" approach. In this case it means that we write code that defines the data model and provides seed data. Then we can run commands to use the code we have written to create the database and populate it with the seed data that we have defined.
The first step here is to create the classes that define the data model. From the root of the project open the "Models" folder and add a new folder called “RetailBanking”. Within the “RetailBanking” folder add a new class called “Customer”. Enter the following code into the “Customer” class:
public class Customer{ public int Id { get; set; }
public string CustomerName { get; set; }
public decimal AccountBalance { get; set; } }
Also within the “RetailBanking” folder add a new class called "RetailBankingDb" and enter the following code into it:
public class RetailBankingDb : DbContext
{
public DbSet Customers { get; set; }
}
If you haven't already you will need to right mouse click on the DbContext class (that our "RetailBankingDb" class inherits from) and choose resolve. This will add a using statement (class reference) for “System.Data.Entity” that is needed for the project to compile without errors.
Now build the solution.
Since we are using "code first" we need to enable code migrations. To do this click on the Package Manager Console (normally at the bottom of Visual Studio) and type the following into the console:
“enable-migrations –contextTypeName RetailBankingDb” and then hit enter.
When we enabled code migrations, the console created a "Migrations" folder at the root of our project which contains a new file called "Configuration". Open the "Configuration" file. We will now edit the "Seed" method of the file to define the seed data. Overwrite the existing code (that has been commented out) and enter the following:
{
context.Customers.AddOrUpdate(p => p.CustomerName,
new Customer { CustomerName = "Andrew Peters", AccountBalance = 50000 },
new Customer { CustomerName = "Brice Lambson", AccountBalance = 100000 },
new Customer { CustomerName = "Andrew Miller", AccountBalance = 5000 }
);
}
Also in the "Configuration" file change the line which says “AutomaticMigrationsEnabled = false” to “AutomaticMigrationsEnabled = true”.
Now the console has permission to create or edit the database if the database doesn't match the structure we defined. Later on if you make any changes to the structure of your "Customer" class this setting gives Entity Framework permission to amend the database to match the structure that you set.
Now build the solution.
In Package Manager Console type “update-database” and hit enter. This will create the database and populate it with the seed data we defined earlier.
To confirm that the seed data has been put into the database we could look in the actual database. If you want to do this open the AppData folder, right mouse click on the database. Then open the Customer table and right mouse click to view the rows of data that were populated.
Its better however to build something programatically that we can also use for testing purposes. To do this we will add some code to the "HomeController.cs" file (the MVC controller) to count the number of records. Then we will display that on the home page ("Index.cshtml").
First, add the following code to the “HomeController.cs” file to create a private method that returns the Customer record count:
private int RecordCount(){ RetailBankingDb context = new RetailBankingDb(); return context.Customers.Count();}
You will also need to right mouse click on RetailBankingDb to resolve and add the using statement to the controller.
Also in the "HomeController.cs file" amend the "Index" method to put a return result from the "RecordCount" method that we just added into the ViewBag. It should now contain the following:
public ActionResult Index(){ ViewBag.RecordCount = RecordCount(); return View();}
In MVC, ViewBag is an object that we can add any property we like to. In this case we added a property called "RecordCount" which we will now display this on the Home page view.
Amend the "Index.cshtml" file so that it shows our ViewBag.RecordCount property:
Now that we know that we have customer data we can amend our Angular App to display the data. Since Bootstrap is also loaded in the Visual Studio MVC template we will use Bootstrap to format the data into a nice table.
JSON (JavaScript Object Notation) is a widely used and efficient standard to output data from a server to the client, particularly JavaScript clients.
MVC and Entity Framework are server side technologies and MVC has built in utilities to efficiently output data in the JSON format. Angular is a client side technology that contains built in capabilities to convert JSON to Html.
So an easy way for Angular and MVC to communicate is to create a server side method that outputs results in the JSON format and call that method from Angular.
Add an MVC controller method that returns a customer list
To create a method on the home controller that returns a customer list in JSON format add the following code to the "HomeController.cs" file:
public JsonResult GetCustomerList(){
RetailBankingDb context = new RetailBankingDb();
return Json(context.Customers, JsonRequestBehavior.AllowGet);
}
We are now going to amend the Angular App so that it calls the method we just put on the MVC controller and gets the list of customers in JSON format.
In the "RetailBankingApp.js" file replace the app.controller method with the following:
app.controller('AccountController', ['$http', function ($http) {
this.hello = "Hello from the Angular Retail Banking Account Controller!";
var accountCtrl = this; accountCtrl.accounts = {};
$http.get('/Home/GetCustomerList').success(function(data){
accountCtrl.accounts = data; }).error(function(data, status){
accountCtrl.error = "Error status : " + status; });
}]);
The above code changes the "AccountController" in our Angular App so that it does the following:
Like most JavaScript libraries including jQuery, Angular can query an Http endpoint and convert a JSON result to Html. We don't have to worry about the intricacies of the conversion.
We define our view using a mixture of Html, CSS and Angular syntax. Within the Html we define a table and utilise Bootstrap classes to format the table with, as the names suggest, a striped look and some hover functionality.
To display our customer list on the home page ("Index.cshtml"), replace the html that displays data from our Angular App with the following:
Run the final output
Run the application using F5. It should look like the following:
Despite some rumours to the contrary we have seen that, for simple layouts at least, its easy to use Angular with MVC, and also to take advantage of the many Microsoft technologies such as Entity Framework 6 which are very easy to use and extremely powerful.
Using AI to build software can result in a slippery slope towards developing average code.
Using DotNet 8 and Aspire With Azure Container Apps Makes Kubernetes Enabled Apps Incredibly Easy
Moving to a micro-services architecture can sometimes seem daunting due to the amount of technical choices to be made and the amount of planning involved. Often however, the migration can be made in manage-able steps. Here is one classic example.
Fast Endpoints have become very popular with .Net developers recently, but how much faster are they than regular Controller endpoints using .Net 8
GPT4 and other tools have good text summarisation capabilities but only Deep Mind is providing the real breakthroughs.
Managing Infrastructure State can be tricky in the cloud. AZTFY and Terraform can help with management