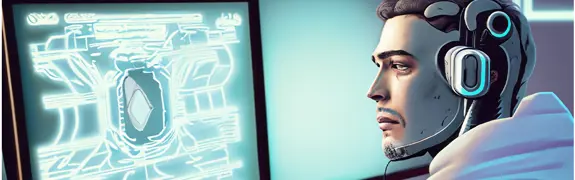
using AI to build software and how to avoid the race to the average
Using AI to build software can result in a slippery slope towards developing average code.
PLEASE ACCEPT OUR. COOKIE POLICY
Whilst routing in Angular is easy to setup, routing for dynamic content in order to maximise search engine visibility is more complex.
There are dozens of articles showing the exact steps involved in setting up basic routing. Therefore, I will just provide sparse instructions for that, and deal in detail with setting up routing for dynamic content.
Firstly, you add the routes in typescript, either in a module.ts file or a routes.ts file eg
{ path: 'article-home', component: ArticleHomeComponent },
This code means that for a route called "article-home", load a component called "ArticleHomeComponent".
Secondly, you add the links in html, usually via a menu as in the following:
This code means that when the user clicks on the "a" tag containing the text "Article", the application will load the route called "article-home" which we defined earlier.
These are the basics. However, if you stop there it means that all of your routes are hard-coded. Therefore, every time you add a page you need to update the routes and the menu or other html links. Most sites will need to go further than this and have dynamic content.
Dynamic content means content can be created for a site, using a C.M.S. or some other system, and the site will reflect that content automatically without any changes to the code in the site being required.
To set up dynamic content we have an entry in the routes that matches the first part of the url with an id at the end:
{ path: 'article-home/:id', component: ArticleHomeComponent },
Then on a certain page the user would see a list of links in a repeating element, for example:
When the above html is rendered the user sees a link (provided by the "a" tag) for each article in the articles collection of the component. When the user clicks on the link the "article-home" route is called, and a value for the "id" parameter is passed to the route object. In this case we are passing in the value of article.articleRef to "id" on the route object. You can see this in the bolded section of the code above.
When the component for the above route is loaded it will need to load the specific content for that url. In this case the content for the article. To do this it will use the "id" parameter provided in the route. We access that value in the following way in our component, usually in the constructor section, assuming we have a variable called "articleRef" in our component:
this.articleRef = this.route.snapshot.params.id;
So on the route object there is a property called snapshot, which has a collection called params which has a property called "id" to which we passed a the value of our articleRef from the article object.
Then we can use the value for "articleRef" to get content for our component in the usual way, for example by calling a service:
this.articleService.getSingle(this.articleRef).subscribe(
x => {
this.article = x;
this.prepareThePageHoweverYouWant();
}
)
The obvious way to set this up would be to just use the id from the database table as the variable to provide to the service. However, this would mean that the url would be something like:
https://yoursite.com/article-home/42
Whilst that would work perfectly well it isn't very good for promoting the content in your site via search engines. Google for example, attaches a certain amount of weight to pages where the subject of the search is contained in the url. Therefore the best way to make your page appear as high as possible in search engine rankings is to use article title as "articleRef". So the url would then become:
https://yoursite.com/article/exact-title-of-page-including-subject
Your component would then pass the title of the page to the service in order to retrieve the content. That obviously means that the article title would have to be a unique key in your database table.
Using this approach to Angular routing your content will appear higher in search engine rankings since the url now contains the subject of the search.
All the best
Using AI to build software can result in a slippery slope towards developing average code.
Using DotNet 8 and Aspire With Azure Container Apps Makes Kubernetes Enabled Apps Incredibly Easy
Moving to a micro-services architecture can sometimes seem daunting due to the amount of technical choices to be made and the amount of planning involved. Often however, the migration can be made in manage-able steps. Here is one classic example.
Fast Endpoints have become very popular with .Net developers recently, but how much faster are they than regular Controller endpoints using .Net 8
GPT4 and other tools have good text summarisation capabilities but only Deep Mind is providing the real breakthroughs.
Managing Infrastructure State can be tricky in the cloud. AZTFY and Terraform can help with management