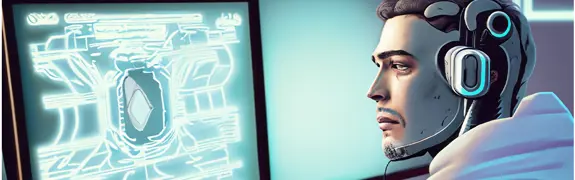
using AI to build software and how to avoid the race to the average
Using AI to build software can result in a slippery slope towards developing average code.
PLEASE ACCEPT OUR. COOKIE POLICY
Often, in a C-sharp program there is a need to return more than one value from a method. For example, if you add a record to a database you might want to return a string value with a success message and an int value with the new record id value.
The long way is to add an object to your project that contains those two properties, and return an instance of that object from your method. The problem with this approach of course is that it adds bloat to the code base and if you have to do it a lot you end up with a lot of objects that are used once or twice.
We have all done it this way but there is a better way which has been around since C# 7.3.
The better way is to return a tuple. If you have the following Class:
public static class DemoClass
{
public static (string, double, int) TupleMethod(string inputStr, double inputDouble)
{
string retString = "Hello " + inputStr;
double retDouble = 1000 * inputDouble;
return (retString, retDouble, 17);
}
}
You can use it as follows:
public class Program
{
static void Main(string[] args)
{
//use a tuple to create values on the fly
var (retString, retDouble, retInt) = DemoClass.TupleMethod("World", 17.94);
Console.WriteLine(retString + " " + retDouble);
//use existing values
string myString;
double myDouble;
int myInt;
(myString, myDouble, myInt) = DemoClass.TupleMethod("World", 17.94);
Console.WriteLine(myString + " " + myDouble + " " + myInt);
}
}
Since Test Driven Development is so popular nowadays, I have used a TDD approach and created a Test project first and then the demo code which you can download here.
Using AI to build software can result in a slippery slope towards developing average code.
Using DotNet 8 and Aspire With Azure Container Apps Makes Kubernetes Enabled Apps Incredibly Easy
Moving to a micro-services architecture can sometimes seem daunting due to the amount of technical choices to be made and the amount of planning involved. Often however, the migration can be made in manage-able steps. Here is one classic example.
Fast Endpoints have become very popular with .Net developers recently, but how much faster are they than regular Controller endpoints using .Net 8
GPT4 and other tools have good text summarisation capabilities but only Deep Mind is providing the real breakthroughs.
Managing Infrastructure State can be tricky in the cloud. AZTFY and Terraform can help with management