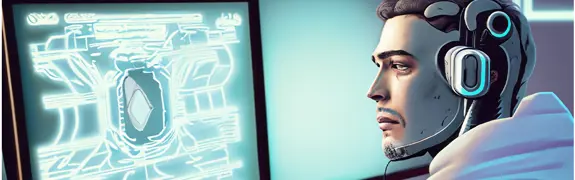
using AI to build software and how to avoid the race to the average
Using AI to build software can result in a slippery slope towards developing average code.
PLEASE ACCEPT OUR. COOKIE POLICY
This article is part of a series which thus far has been on Angular development.
This is the first article on .Net Core development.
Since APIs are most often the way data is delivered to an Angular App, and using an Angular based or mock API quickly becomes more trouble than it is worth, we are now at the point where we have to start implementing a proper API. I am going to use Microsoft Asp.Net Core to develop this. Most of the time there would be a database behind the API, however in this case we won't have one.
To create the project follow these steps :
The API now exists. Run the project (F5) and it should go to the demo endpoint and you will see this:
After the "https://localhost" in the browser you will see the port number allocated by Visual Studio. In my case the port was 44394. It will be different in your case, and for each project you create. This is the port number you will put in your Angular "environments.ts" file when we hook the API content back to the Angular client.
We will now create a class to define the structure of the entities returned by the API. To do this add a file at the project root called "Financials.cs" and paste in the following code, but change the namespace to match your project name:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace{
public class Country
{
public int CountryId { get; set; }
public string CountryName { get; set; }
}
public class Stock{
public int StockId { get; set; }
public string StockName { get; set; }
public int CountryId { get; set; }
}
public class Fund{
public int FundId { get; set; }
public string FundName { get; set; }
public int CountryId { get; set; }
}
public class CountryList{
public List
Countries { get; set; } public CountryList()
{
Countries = new List
(); Countries.Add(new Country() { CountryId = 1, CountryName = "England" });
Countries.Add(new Country() { CountryId = 2, CountryName = "France" });
Countries.Add(new Country() { CountryId = 3, CountryName = "Germany" });
Countries.Add(new Country() { CountryId = 4, CountryName = "Spain" });
Countries.Add(new Country() { CountryId = 5, CountryName = "Wales" });
}
}
}
The API now exists but it has a demo endpoint called WeatherController which we don't want. We will now re-purpose this for our own requirements.
In the Controllers folder right mouse click the "WeatherForecastController.cs" and rename it to "CountryController.cs". This should also rename the class name in "CountryController.cs". Check that it has and if not fix it.
Delete the "Summaries" object at the top of the file and the code that creates it. Paste the following to replace the existing Get Method in "CountryController.cs":
[HttpGet]
public IEnumerable
Get() {
var countryList = new CountryList();
return countryList.Countries;
}
Run the project and go "https:\\localhost:
Delete the "WeatherForecast.cs" file.
We will create a Stock Controller for our API. In the constructor we will use a loop create as many Stock records per Country as we need. To start with 20 records per country is enough. Later on we can change it to see how long it takes using 200, 2000 or 20000 records per country etc.
Copy "CountryController.cs" and paste into the same folder. Rename the new file "StockController.cs". Now edit the "StockController.cs" and change the name of the class to "StockController". Paste in the following code to replace the constructor and the get method:
List
stockList = new List (); public StockController()
{
var countryList = new CountryList();
var countries = countryList.Countries;
stockList = new List
(); for(int i=1; i < 6; i++)
{
Country currentCountry = countries.Where(x => x.CountryId == i).First();
for(int j=1; j<21; j++)
{
stockList.Add(new Stock
{
CountryId = i,
StockId = i * j,
StockName = "Stock " + i * j + " - " + currentCountry.CountryName
});
}
}
}
[HttpGet]public IEnumerable
Get(int countryId) {
return stockList.Where(x => x.CountryId == countryId);
}
Run the project, using the url below (with your port) and you should see the following output for the stock endpoint:
Do the same for FundController (and substitute "fund" for "stock" throughout the class). Run the project and test the Fund endpoint.
We now have a working API. If I have lost you along the way you can access the Github repo here.
We can't connect our Angular App to this API yet as modern browsers won't call an API from a different URL, without that API expressly giving permission. CORS is a way of declaring which clients can access which endpoint in an API.
Using AI to build software can result in a slippery slope towards developing average code.
Using DotNet 8 and Aspire With Azure Container Apps Makes Kubernetes Enabled Apps Incredibly Easy
Moving to a micro-services architecture can sometimes seem daunting due to the amount of technical choices to be made and the amount of planning involved. Often however, the migration can be made in manage-able steps. Here is one classic example.
Fast Endpoints have become very popular with .Net developers recently, but how much faster are they than regular Controller endpoints using .Net 8
GPT4 and other tools have good text summarisation capabilities but only Deep Mind is providing the real breakthroughs.
Managing Infrastructure State can be tricky in the cloud. AZTFY and Terraform can help with management